Setting up your Project
(The Correct Way)
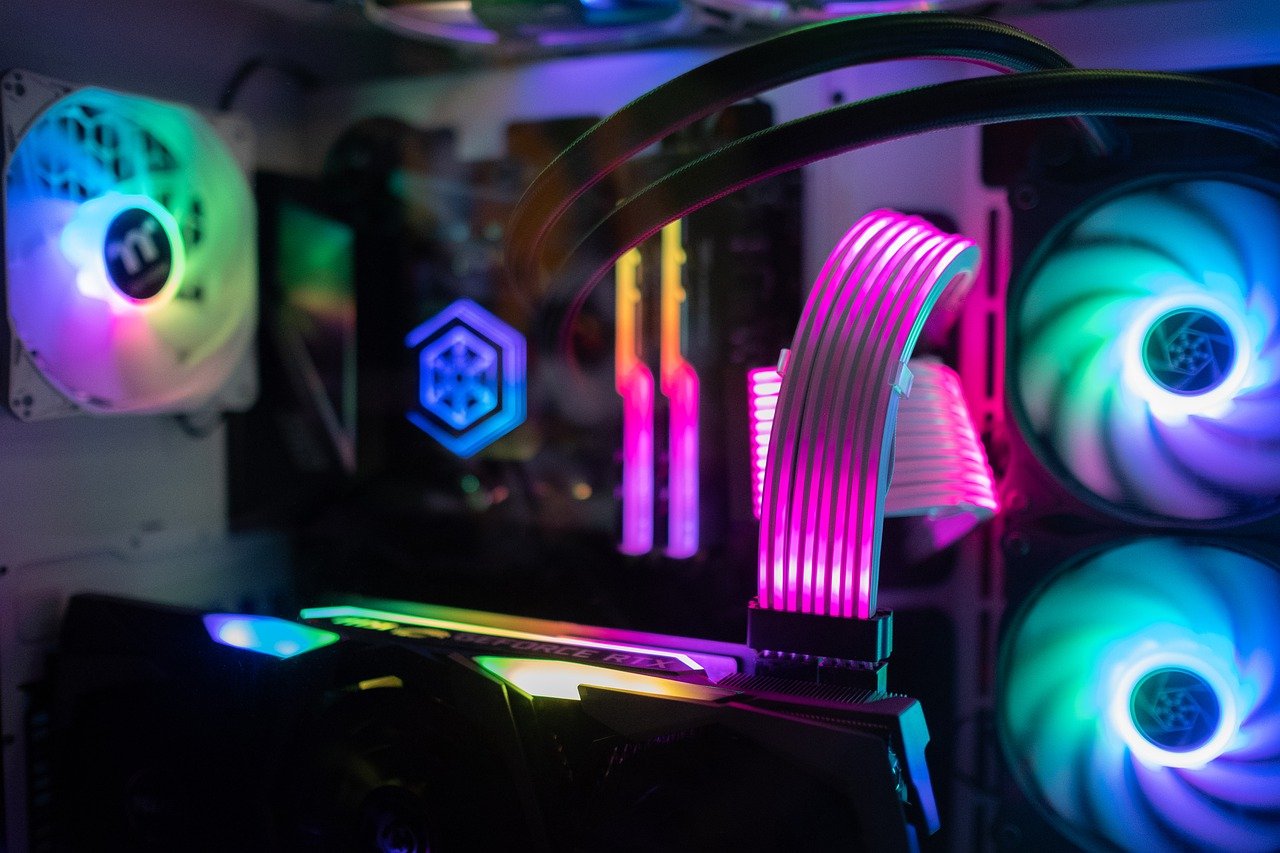
Setting up a project may sound like a simple task to you. However, it is important that we get this step right. By doing so, it will not only impress your technical assessors for your job application, but it will also ensure that your project will remain clean in the long run.
For a short-term project, it is fine just to go to GitHub, create a repository, and call it done. However, in the long run, as more people work on the project, things start to change. As coding style differs, the code becomes harder and harder to read. As a new members join, they might be new to the language, hence using an Integrated Development Environment (IDE) with suggestions will be really helpful to them.
In this Article, I will take you through a very basic project setup using, GitHub, VS Code, Ruby on Rails and Git. You may be using a different stack but similar principles will apply. All you will need is
- A remote origin
- An IDE
- A Code Skeleton in any language
- A version control system
In order to ensure that we keep things focused, the next few articles will only contain a single concept. This will make things easier for people to follow along, it won't be like the Sprint Planning Series where one article has many ideas for a particular phase.
Preparation
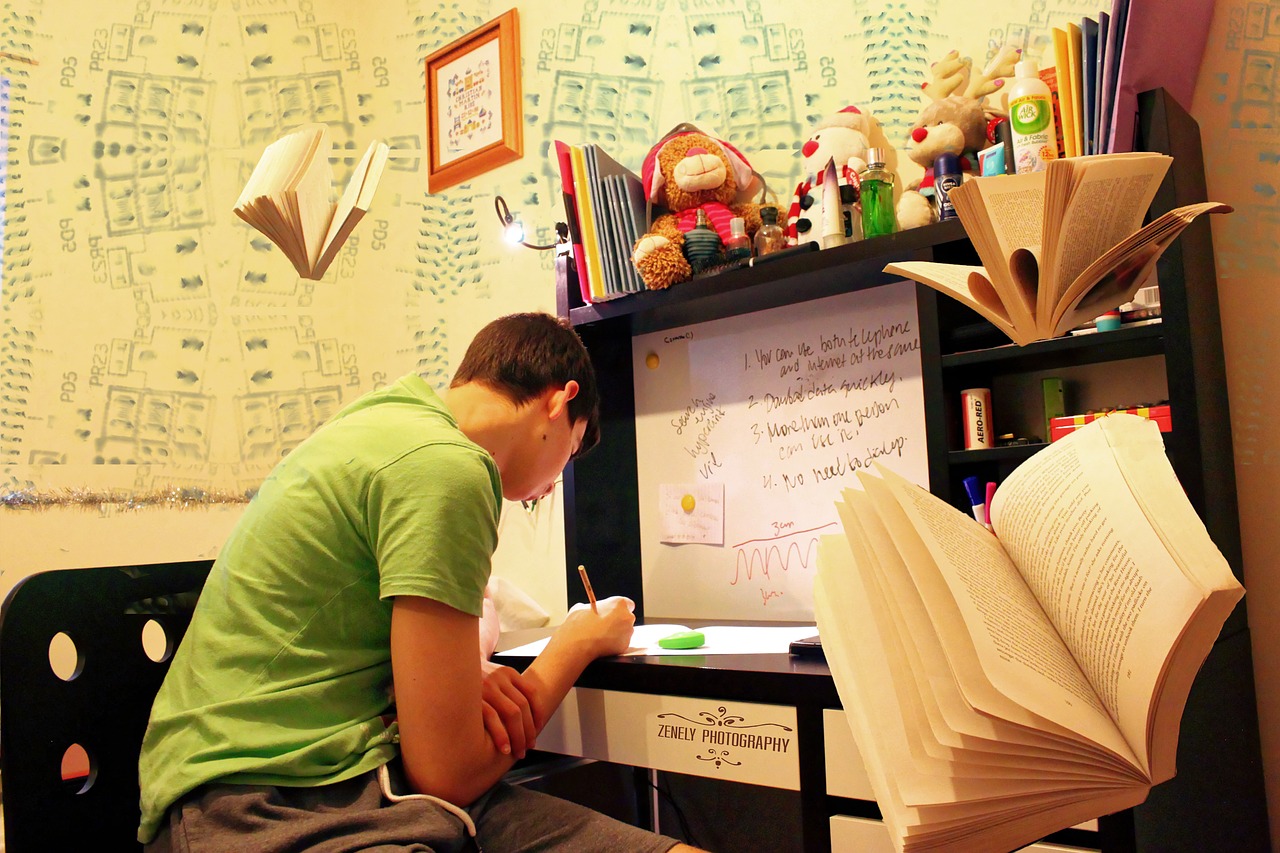
If you want to follow along this project setup series, please setup your computer according to the following website. The setup instructions are courtesy of Le Wagon, I do teach there part time. If you are interested in their courses feel free to sign up. They have offices everywhere world wide.
After that you can open your terminal and copy and paste the following. Replace <YOUR_APP_NAME> with your own application name. If you want you can call it myapp.
rails new \
--database postgresql \
-m https://raw.githubusercontent.com/lewagon/rails-templates/master/minimal.rb \
<YOUR_APP_NAME>
cd <YOUR_APP_NAME>
gh repo create
git push origin main
Setting up VS Code
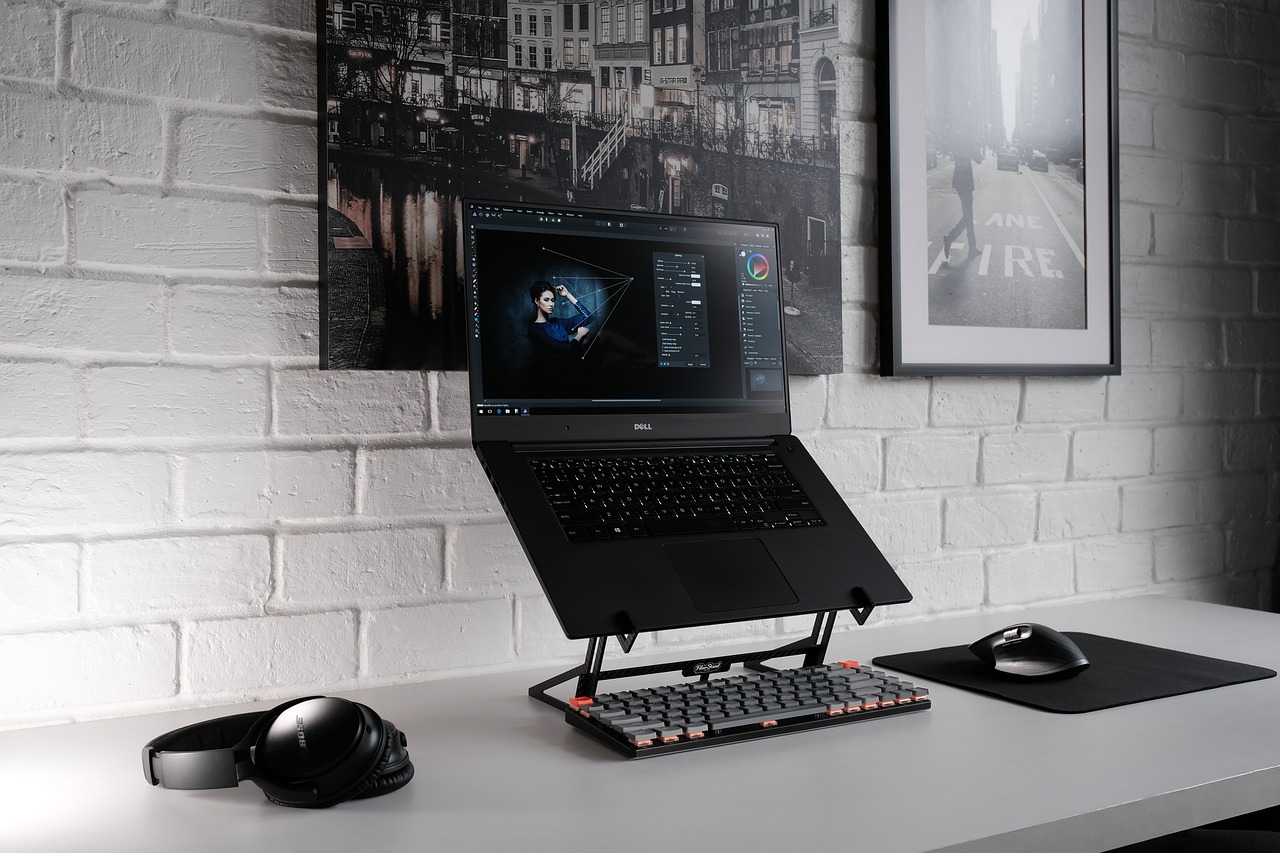
Visual Studio Code is a widely used IDE for any language. Other IDE out there that companies commonly used include Intellij and Eclipse . You can use any IDE you want or prefer, but the concepts remain the same. The first step is to ensure that your IDE is set up for the language that you are going to code. We want to ensure that the setup process is the same for every team member so that when we pair program, things become a lot easier.
For the case of VS Code, it is quite simple as VS Code allows us to add a folder to contain recommended extensions. We can also configure our VS Code really easily through a settings.json file. So long as everybody have the same JSON file, the IDE should remain consistent throughout all team members.
Because we will be using Ruby on Rails, the extensions that I will introduce will be specific to the project. However, if you are using something such as NextJS, NodeJS, GoLang etc. a simple google search will allow you to find the recommended extension.
For Ruby on Rails, there are many extensions that you project could include, however, for simplicity's sake I will introduce to you 5 types which most project will need:
- Code Formatter (esbenp.prettier-vscode)
- Language Beautifier (aliariff.vscode-erb-beautify)
- Language Specific Suggestions (rayhanw.erb-helpers)
- Collaboration Tools (ms-vsliveshare.vsliveshare)
- Version Control Tools (eamodio.gitlens)
A code formatter is used to format our code. This ensures that or coding style remains consistent throughout the project. For example, how we indent the code? Where should the brackets be? Should we end the line with trailing commas? Through the code formatter standardization, this makes the project look as if it was written by one person, which makes things easier to read, learn and write. It is also important that we turn on the format on save feature on, this ensures we don't have to waste time adjusting our coding style to match the required standard.
A language beautifier allows the IDE to colorize the code correctly. Keywords such as const or let should be in a different color when you type them in a js file. Reserved words should also be in a different color to prevent weird variable naming errors. This ensure that the code is easy to read and reduce the chance of dirty code.
A language specific suggester allows the IDE to give suggestions base on what the user is typing. To add on to this, a code generator can also be useful to install. For example when using Eclipse we can easily generate getters and setters when we code in Java. Plugins like these should be installed in every Java IDE to increase productivity.
A collaboration tool allows us to easily collaborate with another user. This makes pair programming and debugging a lot easier.
A version control tool such as gitlens will allow you to easily see who is the author of a particular line of code and when was it changed. This allow you to easily contact the person if there is a need for clarification or explanation.
How to add VS Code Extensions
In the root of your project, you can run the following code to create a VS Code extensions file.
mkdir .vscode
cd .vscode
touch extensions.json
In the extensions.json file, you should add the extensions for your project. For those who are following along, you can copy the file information below
{
"recommendations": [
"rebornix.ruby",
"kaiwood.endwise",
"rayhanw.erb-helpers",
"aki77.rails-db-schema",
"aliariff.vscode-erb-beautify",
"misogi.ruby-rubocop",
"mikestead.dotenv",
"esbenp.prettier-vscode",
"eamodio.gitlens",
"editorconfig.editorconfig",
"ms-vsliveshare.vsliveshare"
],
"unwantedRecommendations": []
}
After creating this file and adding the extensions, you may see VS Code prompting you to download and install some extensions if you haven't got it install. This means that the recommended extensions.json is working.
Format on save
Adjust your VS Code settings code > preferences > settings, add a , at the end of the file and paste the following code
{
...
"editor.bracketPairColorization.enabled": true,
"editor.defaultFormatter": "esbenp.prettier-vscode",
"editor.formatOnSave": true,
"editor.codeActionsOnSave": {
"source.fixAll": true
},
}
If there is a yellow squiggly line in your settings.json, most likely the code is repeated. Try to combine them or remove the duplicates.
Conclusion
To conclude, setting up a project is easy, doing it correctly it is hard. It is also important that we maintain a document on how we should setup a project and its configurations to make the onboarding process easier. This document will change overtime as a project grows, new plugins are introduced or removed to increase the code quality or increase productivity.
Next Article: The Git Workflow coming on 21/03/2022
Last Updated: 16/03/2022